Are you looking to showcase solely the parent category for your WordPress posts?
Typically, WordPress themes exhibit all categories linked to a post. Nevertheless, certain users may prefer to exhibit solely the parent category and exclude any child categories.
This article will guide you on how to alter the WordPress post loop to showcase only the parent category on a single post.
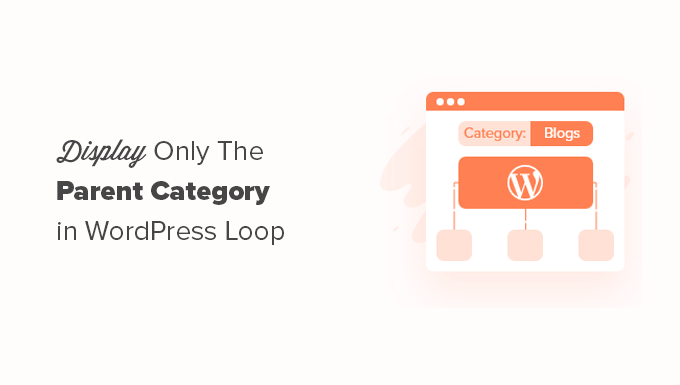
When to Show Only the Parent Category in WordPress
Many website owners utilize parent and child categories as a means of structuring their websites. This approach involves grouping related content into categories, with parent categories serving as the overarching themes and child categories providing more specific subtopics.
For example, a travel blog may organize its content by travel destinations, with each region serving as a parent category and individual cities as child categories. This hierarchical structure allows for easy navigation and helps users quickly find the information they are looking for.
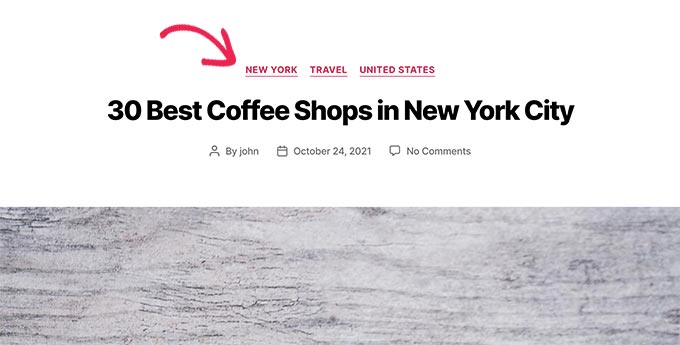
In a similar manner, a food blog might categorize their recipes into parent and child categories. For example, the parent category could be based on cuisine type, while the child category could be based on dish type.
Typically, WordPress themes utilize the_category() template tag to showcase all categories linked to a post. While this function is effective, it will display all categories in alphabetical order and disregard the parent/child hierarchy.
With that said, let’s explore how to modify this behavior and solely exhibit the parent category within the WordPress loop.
Display only the parent category in the WordPress post loop
To begin, you must insert the subsequent code into the functions.php file of your theme or utilize a code snippets plugin like WPCode (which is highly recommended):
functionwpb_get_parent_terms($taxonomy= ‘category’)
{
$currentPost= get_post();
$terms= get_the_terms($currentPost->ID, $taxonomy);
if(is_wp_error($terms)) {
/** @var \WP_Error $terms */
thrownew\Exception($terms->get_error_message());
}
$map= array_map(
function($term) use($taxonomy) {
return'<a href=”‘. esc_url(get_term_link($term->term_id,
$taxonomy)) . ‘” title=”‘. esc_attr($term->name) . ‘”>
‘ . $term->name . ‘
</a>’;
},
array_filter($terms, function($term) {
return$term->parent == 0;
})
);
returnimplode(‘, ‘, $map);
}
This code generates a new function named wpb_get_parent_terms(). By default, this function solely exhibits parent categories.
Subsequently, you must insert this function into your WordPress theme files at the desired location to display only the parent category.
To determine the appropriate template file, refer to our WordPress template hierarchy cheatsheet for beginners.
Essentially, you will need to locate the the_category(); template tag within the WordPress loop. Once located, you will need to substitute it with the subsequent code:
<?php wpb_get_parent_terms(); ?>
This code will only show your top-level category.
If you have several categories that are top-level or independent, then all of them will be shown as well.
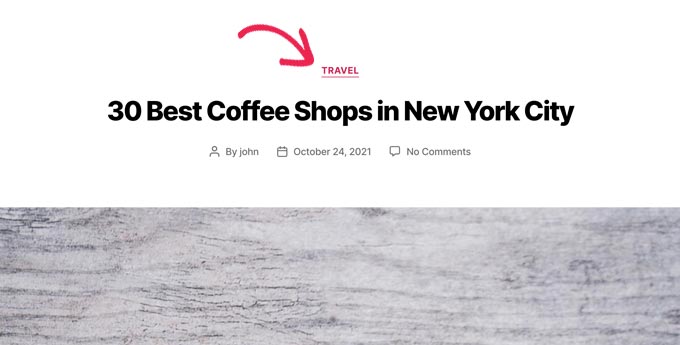
You can use the same code snippet for other taxonomies as well, such as WooCommerce product categories or any custom taxonomy that you have created.
To do so, you just need to make some modifications to the code, like this:
<?php wpb_get_parent_terms( ‘product_cat ‘); ?>
This code snippet is designed to showcase product categories specifically for a WooCommerce store.
Additionally, it will only display the top-level or independent categories for a product.
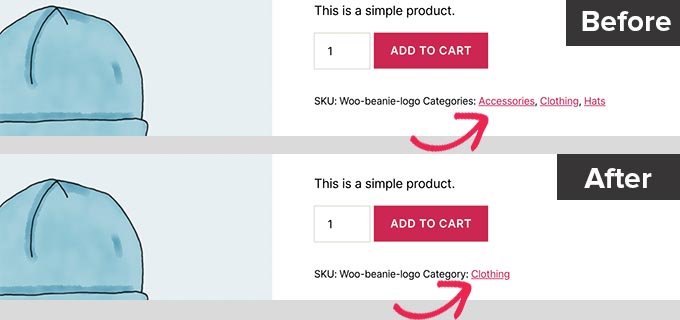
To display a custom taxonomy, simply replace “product_cat” with the name of your custom taxonomy.